If you develop websites, you’ve probably heard of TypeScript by now. TypeScript is a superset of JavaScript that allows developers to write code that is more predictable and easier to debug, and makes it easier to refactor and maintain large codebases.
No wonder it was (overwhelmingly) voted the most used flavour of JavaScript in the 2021 State of JS survey. Current levels of TypeScript usage are through the roof, and with good reason. But if you live with Patrick Star under a rock in the bottom of the ocean and don’t know anything about TypeScript, fear not! This article will cover everything you need to know.
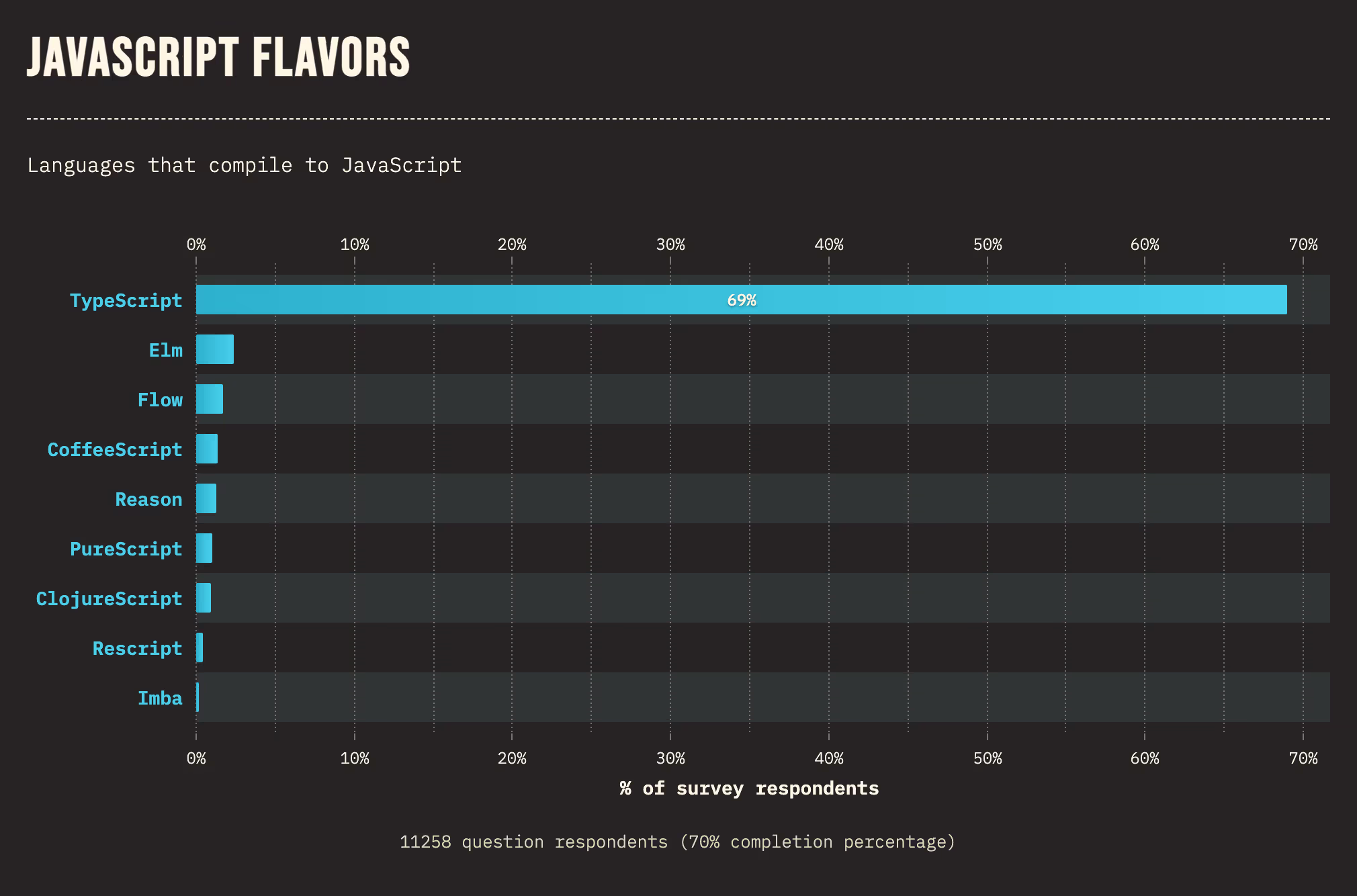
What is TypeScript?
TypeScript is a free and open source programming language that was developed and is maintained by Microsoft. Put simply, TypeScript is a superset of JavaScript that turns it into a statically-typed language. Okay, maybe that wasn’t put that simply so let's break down that statement.
TypeScript is a superset of JavaScript that turns it into a statically-typed language.
Being a superset of JavaScript means that TypeScript contains all the rules that define JavaScript, and more. That is, all valid JS code is also valid TS code. This makes it backwards-compatible with JS and incredibly easy to adopt since all your ugly JS code is already valid TS code.
Can browsers run TypeScript natively? Well, no. Like any other JavaScript flavour, you have to compile your TypeScript code into JS code—that's done by the TypeScript Compiler, tsc. But, speaking of being backwards-compatible, most of the JavaScript applications created today go through some kind of compilation, bundling, or minification process before it goes live, so compiling your TypeScript code is just one more step on that workflow. That also means that your TypeScript code never sees the light of day—by which I mean you never push it to production. The code that goes live is never TypeScript code, but the resulting JavaScript code that you compiled.
Now, what do I mean by TS turning JS into a statically-typed language? When it comes to typing, programming languages can be either statically-typed or dynamically-typed. In statically-typed languages, variables created to store numbers can only ever store numbers and, likewise, variables created to store strings can only store strings. That is, once declared, variables can’t change types.
Dynamically-typed languages, on the other hand, allow variables to change types. That is, a variable that stores numbers can also store strings, objects, and arrays. That can sound a bit chaotic, but dynamically-typed languages offer a level of flexibility that is very useful in certain applications.
JavaScript is a dynamically-typed language, along with PHP, Python, and Ruby. These are all popular and important languages in the programming world. When it comes to JavaScript, however, as with many things in life, you can have too much of a good thing.
![A meme depicting the fact that, in JavaScript, 0 == "0" is true and 0 == [] is also true, but "0" == [] is false](https://cdn.prod.website-files.com/6712af348887efb8c3396c9c/671acdd53837f3b645cb8ac9_JS%2520Patrick%2520Star_0.avif)
Why use TypeScript?
Programming languages can be placed on a spectrum from strict to flexible. Being too strict can be too restrictive and force you to write verbose and unnecessarily complicated code (Java, I’m looking at you), whereas having too much flexibility can feel like walking through a minefield because the language is too wild and unpredictable. This last one is JavaScript, so we use TS to tame JS.
TypeScript will catch silly errors while you’re writing the code. Without TypeScript, these silly errors can build up and join forces to create the ultimate boss bug in production. So you might think adding types to your code is a pain—and sometimes it really is—but you know what is even more of a pain? When you're trying to access an optional property in an object and forget to use optional chaining. In all your local tests that property might be present and you never caught the error, but since software development extends Murphy's Law, anything that can go wrong will go wrong. So you push your app to production, and the very second a user interacts with it, everything explodes and there's chaos in the streets and cars are set on fire, and a mere five years later we're surviving in small groups of hunter-gatherers telling tales about a time when something called "the internet" existed, before that person made that stupid JavaScript mistake. Do you want to be responsible for the end of society as we know it? I didn't think so.
Maybe I'm being overly dramatic, but I honestly think that taking the extra time to make sure this kind of thing can't possibly happen is the responsible way to do our jobs.
Typing also makes the code a lot more readable and easier to maintain. We have all written code that we revisit a few months later to just stare at the screen for hours, clueless about what's going on in it. Not to mention the poor souls that will have to work on your code in the future. If you can't even decipher what your cryptic code is doing, how do you expect someone else to?
Using TypeScript cuts all the maintenance hours you would spend just figuring out the shape of the data you’re working with.
All of that takes time, and time, my friends, is money. Using TypeScript cuts all the maintenance hours you would spend just figuring out the shape of the data you’re working with, while also being an elegant way of documenting the code. It’s much easier to understand a piece of code when you can just hover any of the variables involved and see their types and I don't see any reason not to use these features. Although, some people just want to watch the world burn. I get it. Hopefully you're not one of those.
In addition to all these features, TypeScript also offers support for the latest versions of JavaScript, including ECMAScript 6 (ES6) and ECMAScript 7 (ES7), as well as JSX syntax for React! This means that developers can use the latest and most powerful features of JavaScript while still benefiting from the static typing and other features offered by TypeScript. I don't want to be too extreme here, but you still prefer not to use TypeScript after learning about all these advantages, then I have to call you a dummy. Sorry to be so harsh, but you can't fight the facts. Well, you can, but you'll lose.
The bottom line is using TypeScript means you’re in control of the code, not the other way around.
How to use TypeScript
Most frameworks today have a boilerplate option that includes TypeScript. Want to create a React application with TypeScript? There's a template for that. A Vue application? There's a template for that. An Angular application? Well, with Angular you don't even have an option because it uses TypeScript by default.
Just to get the hang of it and illustrate how simple it is, I will show you how to create your first TS application. Please note, I'm using yarn as a package manager here, but you can use npm if you prefer.
Let's start by creating an empty project. You can do that by heading to any empty folder (the folder doesn't have to be empty but things might get messy if it's not) and use the following command:
yarn init -y
This will create a package.json file. I'm using the -y parameter because the init command asks you for information about the project, and that parameter will confirm all of the suggestions without you having to answer them.
With that done, let's add TypeScript to our project.
yarn add -D typescript
This command will create our beloved node_modules folder and install TypeScript as a dev dependency. "Why a dev dependency?" you're asking, and as the wizard master of the magical arts of the internet that I am, I hear you. As I mentioned earlier, your TypeScript code will never go live. What goes live is the JavaScript code that is compiled from your TS code. That means the TypeScript package isn't actually needed for the application to run, so it's not really a production dependency. As a bonus, since TypeScript is never bundled with the rest of the application, it doesn't really affect the size of your app.
Now that we've done that, let's create a file called hello.ts in your folder. Here's the content of my hello.ts file:
// hello.ts
const hello = (name: string) => {
return `Hello, ${name}!`;
};
hello('Patrick');
So let's analyze this code. I'm using Visual Studio Code, by the way.
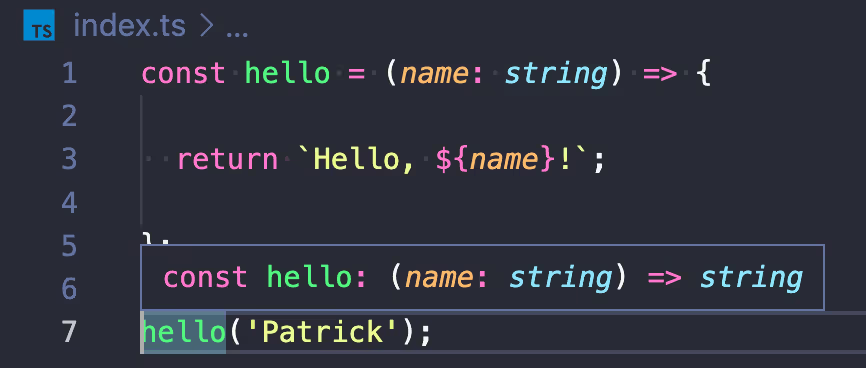
The first thing to notice is I have the colon and the keyword string. That is telling my code that the argument name is of the type string. That's the only difference if you compare this snippet to JavaScript code but... what? What happens when I hover the line where my function is being called? Oooh, look at that function signature! Hmmm delicious! I didn't even type the function's return, but TypeScript is inferring the type and letting me know that hello is a function that receives a string argument called name, and that it returns a string.
Now let's see what happens if I try to give my function a number instead of a string.

Wait, what's that, TypeScript? The argument of type 'number' is not assignable to parameter of type 'string'? Wow! Thanks for letting me know!
This is a very silly example, but hopefully it's enough for you to see the power of this tool. It tells you a lot more about the code that you're working with. Imagine your co-worker wrote that hello function, not you, or that that function is actually part of a third-party library. By knowing it accepts one argument of the type string and that it also returns a string, you have a much better understanding about the code than you would have if you weren't using TypeScript.
Not only that, but if you made a mistake and ended up passing that function as a parameter of the wrong type, TypeScript will let you know before you cause the apocalypse. Oh, these are not enough reasons? Alright, check this out:

If I add a dot after the function, Visual Studio Code will let me know what properties or methods are available from there. Since it knows this function returns a string, it's showing me properties (like length) and methods (like replace and split) that are available in string objects. Because it knows! How useful is that? You don't even need to check what you're working with; it will tell you. And if you do something wrong, it will let you know. Basically, TypeScript is the ultimate companion for JavaScript developers (second only to AI, but let's not get into that just yet).
Basically, TypeScript is the ultimate companion for JavaScript developers (second only to AI, but let's not get into that just yet).
So, for a final step, you must be asking yourself, "how do I compile this thing?" For this example, you can compile your code using the following command:
yarn tsc hello.ts
I'm using yarn before tsc because I installed TypeScript locally to this project, and this command will access the compiler from inside the node_modules folder. This command will create a new file called hello.js in your current directory, which contains the compiled JavaScript code for your TypeScript function. That's all there is to it!
This is just the tip of the iceberg when it comes to TypeScript, and if I did my job right, you've learned it's an extremely powerful tool when it comes to helping you produce quality JavaScript code. TypeScript is a fantastic superset of JavaScript that is easy to learn, use, and maintain. If you're looking to improve the quality and reliability of your code, give TypeScript a try! Satisfaction is guaranteed.